ALL >> Education >> View Article
Essential Techniques For Mastering Command Line Arguments In C
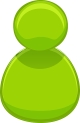
Understanding Command Line Arguments
Command line arguments are values that are passed to a C program when it is executed from the command line. They are typically used to provide input data, configure program settings, or select specific functionalities.
Key Concepts:
argc: This integer variable contains the entire amount of command-line arguments supplied to the program, including the program name itself.
argv: This array of strings contains the actual command line arguments. The first element (argv[0]) always holds the name of the program.
Passing Command Line Arguments
To accept command-line arguments in your C program, you must edit the main function declaration to include the argc and argv parameters.
C
int main(int argc, char *argv[]) {
// ...
}
Accessing Command Line Arguments
You can access the command line arguments using the argv array. The array index corresponds to the argument's location. For example, argv[1] refers to the first argument after the program name.
Example:
C
#include
int main(int argc, char *argv[]) ...
... {
if (argc < 2) {
printf("Usage: %s \n", argv[0]);
return 1;
}
printf("First argument: %s\n", argv[1]);
printf("Second argument: %s\n", argv[2]);
return 0;
}
Practical Applications
Command line arguments are widely used in various C programming scenarios, including:
File processing: Passing filenames as arguments to read or write data.
Configuration settings: Specifying program options or parameters.
Data input: Providing input values directly from the command line.
Debugging and testing: Debugging and testing include controlling program behavior for testing reasons.
Advanced Topics in Command Line Arguments
1. Option Flags:
Use option flags to supply more information or to modify program behavior.
Common option flags include -h, --help for usage information, -v, --verbose for verbose output, and -c, --config for specifying configuration files.
2. Optional Arguments:
Allow for arguments that are optional and may or may not be present.
Use conditional logic to check for the presence of optional arguments and handle them accordingly.
3. Default Values:
Provide default values for arguments that are not specified.
This can improve user experience and make your program more robust.
Example:
C
int num = 10; // Default value
if (argc > 1) {
num = atoi(argv[1]);
}
4. Argument Parsing Libraries:
For complex command-line interfaces, consider using libraries like getopt or argparse to simplify argument parsing.
These libraries provide features like automatic help generation, error handling, and validation.
5. Positional Arguments:
Positional arguments are arguments that are expected in a specific order.
Use conditional logic to check the correct number of positional arguments and handle errors if necessary.
6. Custom Argument Parsing:
For more complex scenarios, you can implement your own custom argument parsing logic.
This gives you full control over how arguments are processed.
7. Combining Arguments:
You can combine positional arguments and option flags in various ways to create flexible command-line interfaces.
Consider combining the two to create a clear and straightforward user experience.
By mastering these advanced topics, you can create sophisticated and user-friendly command-line interfaces for your C programs.
Beyond the Basics
In addition to the fundamental concepts, here are some advanced considerations when working with command line arguments:
Error Handling: Implement proper error handling to gracefully handle situations where incorrect or missing arguments are provided.
Argument Parsing: For complex command-line interfaces, consider using libraries or custom functions to parse arguments efficiently.
Option Flags: Use option flags (e.g., -h, --help) to provide usage information or enable specific program features.
Optional Arguments: Allow for optional arguments that can be specified or omitted.
Default Values: Provide default values for arguments that are not specified.
Conclusion
Mastering command line arguments is a valuable skill for C programmers. By understanding how to effectively use them, you can create more flexible, user-friendly, and powerful applications.
For More Details Visit : C Language Online Training
Register For Free Demo on UpComing Batches : https://nareshit.com/new-batches
Naresh IT is a beacon of excellence in the world of software training, illuminating the path to success forcountless aspiring IT professionals. With a commitment to quality education and hands-on learning, Naresh IT empowers individuals to unlock their potential and achieve their career goals. Join the Naresh IT community and embark on a transformative journey towards a brighter future in the dynamic field of technology. For More Details Visit : https://nareshit.com/courses/c-language-online-training Register For Free Demo on UpComing Batches : https://nareshit.com/new-batches
Add Comment
Education Articles
1. How Exercise Books Foster Organization And CreativityAuthor: The School Print Company
2. Diy Vs. Professional: Choosing The Right Approach For Your School Logo
Author: The School Print Company
3. Top-rated Icse High School In Bhopal For Quality Education
Author: Ronit Sharma
4. Best B. Tech And Engineering College In Meerut And Up
Author: CONTENT EDITOR FOR SAMPHIRE IT SOLUTIONS PVT LTD
5. The Five Borough Academic: Cross-cultural Research In Nyc's Diverse Communities
Author: jonesmiller
6. The Future Of Advertising: How Cgi Is Replacing Traditional Commercials
Author: Rajat Sancheti
7. What Is Iso 27001 Procedures And Why Do They Matter?
Author: john
8. Devops Foundation Certification Course
Author: Simpliaxissolutions
9. The Best Gcp Devops Online Training Institute In Bangalore
Author: visualpath
10. Best Hotel Management College In Delhi
Author: harsh thapa
11. Servicenow Online Training | Servicenow Course In India
Author: krishna
12. Best Google Cloud Platform Ai Training In Hyderabad
Author: visualpath
13. Aws Data Engineering Online Training | Data Analytics
Author: naveen
14. Oracle Fusion Cloud Hcm | Oracle Fusion Hcm Training In Pune
Author: visualpath
15. Why Choose The Certified Big Data Foundation? Key Certification Objectives Explained
Author: gsdc