ALL >> Computer-Programming >> View Article
"mastering Python: A Comprehensive Guide For Aspiring Programmers"
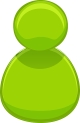
Introduction
Python has gained immense popularity due to its simplicity, readability, and vast array of libraries. It is widely used in web development, data analysis, artificial intelligence, scientific computing, and more. This guide aims to provide a thorough understanding of Python programming, making it accessible for both beginners and seasoned programmers.
1. Getting Started with Python
Installing Python
Before you can start coding, you need to install Python on your computer. Visit the official Python website, download the installer for your operating system, and follow the instructions to install it.
Writing Your First Python Program
Once installed, you can write your first Python program. Open your text editor, type the following code, and save the file with a .py extension:
python
Copy code
print("Hello, World!")
Run the program by opening a terminal or command prompt, navigating to the directory containing your file, and typing:
sh
Copy code
python filename.py
You should see the output:
Copy code
Hello, World!
...
... 2. Basic Python Syntax
Variables and Data Types
Python supports various data types, including integers, floats, strings, and booleans. You can assign values to variables without explicitly declaring their type:
python
Copy code
x = 10
y = 3.14
name = "Alice"
is_active = True
Control Structures
Python uses indentation to define code blocks. Common control structures include if statements, for loops, and while loops:
python
Copy code
# If statement
if x > 5:
print("x is greater than 5")
# For loop
for i in range(5):
print(i)
# While loop
count = 0
while count < 5:
print(count)
count += 1
3. Functions and Modules
Defining Functions
Functions are reusable blocks of code that perform a specific task. You can define functions using the def keyword:
python
Copy code
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
Importing Modules
Python has a rich standard library and supports third-party modules. You can import modules using the import keyword:
python
Copy code
import math
print(math.sqrt(16))
4. Working with Data Structures
Lists
Lists are ordered collections of items that can be of different types:
python
Copy code
fruits = ["apple", "banana", "cherry"]
print(fruits[1]) # Output: banana
Dictionaries
Dictionaries store key-value pairs, providing an efficient way to retrieve values based on keys:
python
Copy code
person = {"name": "Alice", "age": 30}
print(person["name"]) # Output: Alice
5. File Handling
Python makes it easy to work with files. You can open, read, write, and close files using built-in functions:
python
Copy code
# Writing to a file
with open("example.txt", "w") as file:
file.write("Hello, World!")
# Reading from a file
with open("example.txt", "r") as file:
content = file.read()
print(content)
6. Object-Oriented Programming (OOP)
Python supports OOP, allowing you to define classes and create objects:
python
Copy code
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
return f"{self.name} says woof!"
my_dog = Dog("Buddy", "Golden Retriever")
print(my_dog.bark())
Conclusion
Mastering Python opens up numerous possibilities in various fields. This guide covers the basics and introduces you to advanced concepts, providing a strong foundation for your programming journey. Keep practicing and exploring Python's vast ecosystem to unlock its full potential.
Add Comment
Computer Programming Articles
1. Top Ai Development Company In Delhi: Leading Artificial Intelligence Services By DoubleklickdesignAuthor: Prince
2. What Are The Best Coding Institutes In Bhopal?
Author: Shankar Singh
3. Innovating Blockchain Strategies With Mev Bot Technology
Author: aanaethan
4. How To Choose The Right Coding Institute In Bhopal
Author: Shankar Singh
5. Streamline Your Finances With The Best Bookkeeping Software In Zambia
Author: Doris Rose
6. Maximizing Ebay Success With Maropost/neto Partnerships
Author: rachelvander
7. The Rise Of Ai In Modern Gaming
Author: Saira
8. Enhancing Business Efficiency With Entrust Network: Singapore’s Premier It Solutions Partner
Author: Entrust Network Services
9. Ai And Ml Training: Empowering Your Career With Infograins Tcs
Author: Infograins tcs
10. How To Evaluate Coding Institutes In Bhopal?
Author: Shankar Singh
11. Revolutionizing Delivery Services With Application Development
Author: basheer ansari shaik
12. How Google Cloud Platform Aids Businesses And Keeps Its Data Safe?
Author: Stuart
13. Custom Web Development Solutions In Surat For Growing Businesses
Author: sassy infotech
14. Video Streaming App Development: 12 Key Features, Architecture And Cost
Author: Byteahead
15. Understanding Google Analytics Events
Author: Byteahead