ALL >> Computer-Programming >> View Article
Mastering Go: Guide To Type Declarations, Variables, And Constants
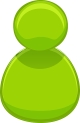
Constants in Go
In Go, constants are fixed values that are known at compile time and remain unchanged throughout the program’s execution. They are defined using the const keyword and can be of basic types like integers, floats, strings, and booleans. Constants help improve code readability and maintainability by ensuring certain values remain consistent.
Declaring Constants
Constants are declared using the const keyword, followed by the constant's name, type, and value. The type can often be omitted because Go infers it from the assigned value.
const Pi float64 = 3.14159
const Greeting = "Hello, World!"
const DaysInWeek = 7
const IsTrue = true
Grouping Constants
You can group constants using parentheses, which is useful for organizing related constants.
const (
Pi = 3.14159
Greeting = "Hello, World!"
DaysInWeek = 7
)
Untyped Constants
Go allows untyped constants, which do not have a fixed type until they are used in a context that requires a specific type. This provides flexibility.
const Pi = 3.14159 // untyped ...
... constant
var radius float64 = 10
var circumference = 2 * Pi * radius // Pi is used as a float64 here
Enumerated Constants
Enumerated constants are a way to create a sequence of related constants, often using the iota keyword, which simplifies creating incrementing values.
const (
Sunday = iota // 0
Monday // 1
Tuesday // 2
Wednesday // 3
Thursday // 4
Friday // 5
Saturday // 6
)
The iota keyword represents successive integer constants starting from 0 and resets to 0 whenever the const keyword appears.
Constants with Expressions
Constants can be defined using expressions as long as the result is a compile-time constant.
const (
SecondsInMinute = 60
MinutesInHour = 60
HoursInDay = 24
SecondsInDay = SecondsInMinute * MinutesInHour * HoursInDay // 86400
)
Limitations of Constants
Immutable: Constants cannot be changed once defined.
Compile-Time: The value of a constant must be known at compile time.
Basic Types Only: Constants can only be of basic types. More complex types like slices, maps, and structs cannot be constants.
Practical Usage of Constants
Constants are often used to define values that are used multiple times in the code, such as configuration settings, fixed dimensions, or commonly used strings.
package main
import "fmt"
const (
Pi = 3.14159
AppName = "MyApp"
MaxUsers = 100
WelcomeMsg = "Welcome to MyApp!"
)
func main() {
fmt.Println(AppName, "allows up to", MaxUsers, "users.")
fmt.Println(WelcomeMsg)
fmt.Printf("The value of Pi is approximately %.2f\n", Pi)
}
Add Comment
Computer Programming Articles
1. Which Institute Is Best For Coding And Programming In Bhopal?Author: Shankar Singh
2. Top 9 Benefits Of Custom Mobile Application Development
Author: Byteahead
3. Top 10 Creative Business Ideas For Entrepreneurs
Author: Byteahead
4. Top 10 Apps Like Tiktok Everyone Should Check Out
Author: Byteahead
5. Is The Apple Watch Series 7 Worth It For Seniors?
Author: Ashish
6. The Ultimate Guide To Ebay Product Listing Services: Elevate Your Online Store
Author: rachelvandereg
7. Which Are The Best Java Coding Classes In Bhopal?
Author: Shankar Singh
8. Warehouse Management In Zambia: Essential Features To Look For
Author: Doris Rose
9. Ecommerce Web Design And Development In Melbourne With The Merchant Buddy
Author: themerchantbuddy
10. Why Website Maintenance Is Crucial For Business Success
Author: Yogendra Shinde
11. Boost Your Business With Smart Invoice Pos Software In Zambia
Author: Cecilia Robert
12. How Stablecoin Development Ensures Stability And Security?
Author: Michael noah
13. Công Cụ Tính Chiều Cao Chuẩn Từ Minbin Tool: Đo Lường Và Cải Thiện Chiều Cao Hiệu Quả
Author: KenJi123
14. How To Make A Courier App For Courier Delivery And Tracking Service
Author: Deorwine Infotech
15. Reputation Management In The Digital Age: Protecting And Enhancing Your Law Firm’s Image
Author: jamewilliams